Algorithm for file updates in Python - Google Cybersecurity Certificate (Lab Assignment)
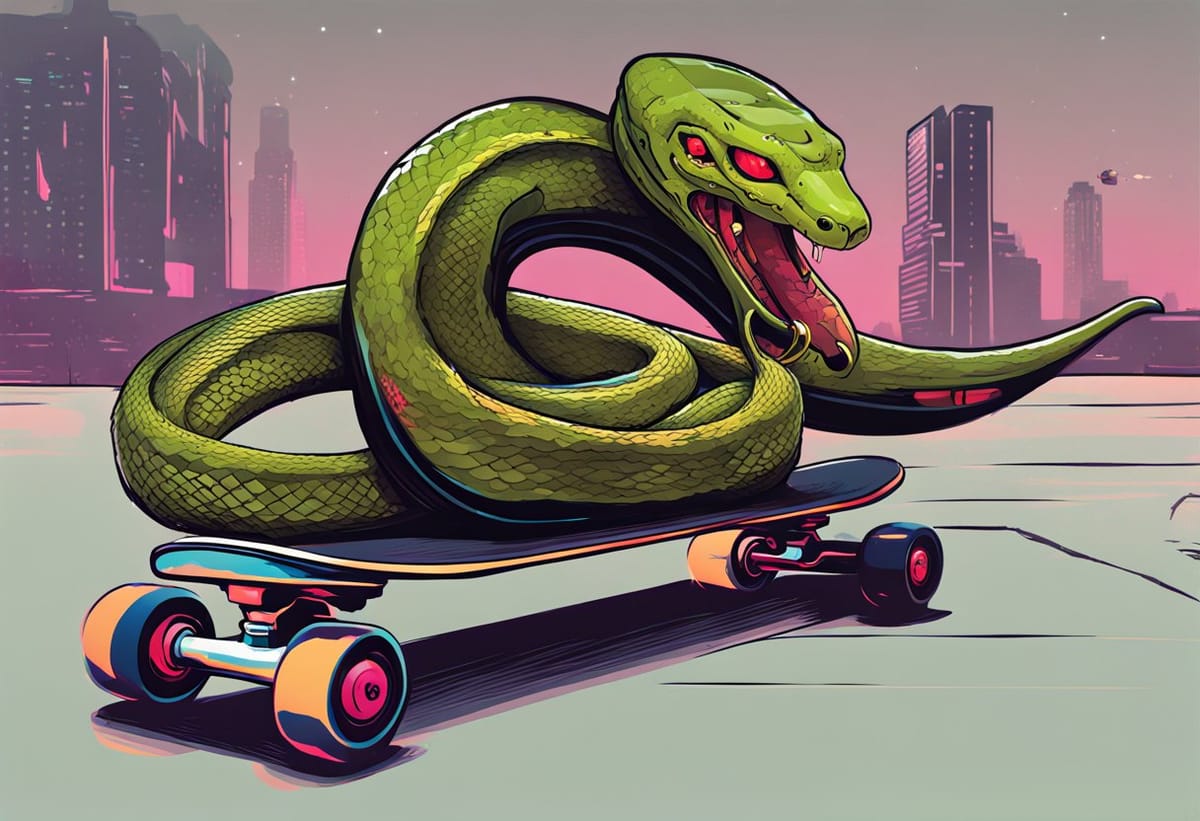
Project description
Our goal for this project is to practice developing algorithms and file read/write in Python3.
Given scenario:
You are a security professional working at a health care company. As part of your job, you’re required to regularly update a file that identifies the employees who can access restricted content. The contents of the file are based on who is working with personal patient records. Employees are restricted access based on their IP address. There is an allow list for IP addresses permitted to sign into the restricted subnetwork. There’s also a remove list that identifies which employees you must remove from this allow list.
Your task is to create an algorithm that uses Python code to check whether the allow list contains any IP addresses identified on the remove list. If so, you should remove those IP addresses from the file containing the allow list.
Open the file that contains the allow list
First, we need to assign the file containing the allow list to a variable. Then, we can open the file with read permissions.
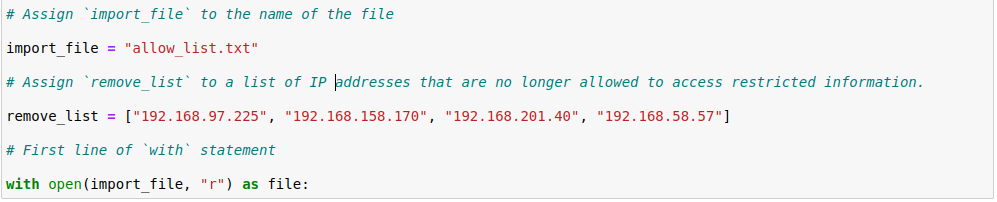
Read the file contents
Then, we can read the file contents to see what is in the allow list. To read the file contents, we use the with open(filename, “r”) as file then the .read() function on the file:
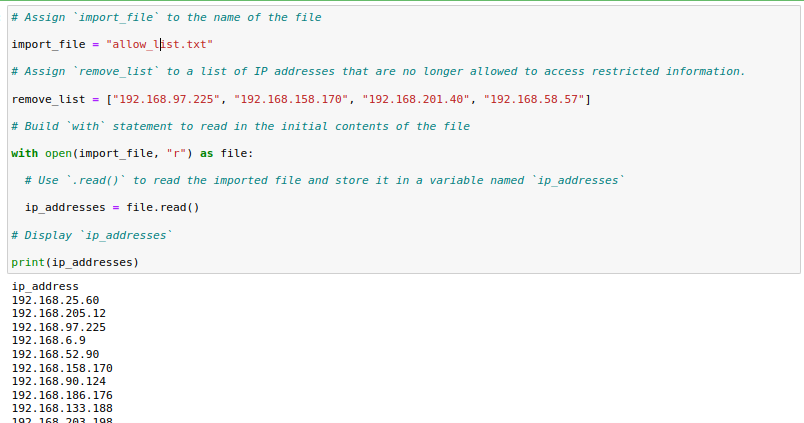
Convert the string into a list
Next, we need to convert this variable into a list so that we can compare it to the remove_list list. By default, split() will split on whitespace so we will use that in this case:
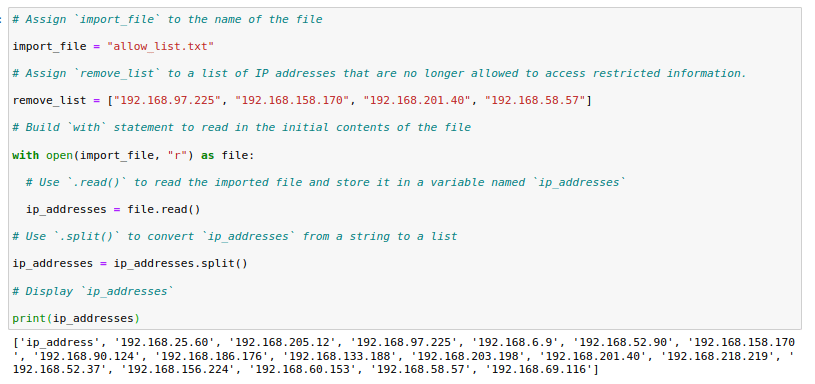
Iterate through the remove list
In order to compare all of the IP addresses, we will need to iterate through the remove list using a for loop. So, we will loop through the values of our ip_addresses and in the next step compare each one to the remove_list values.
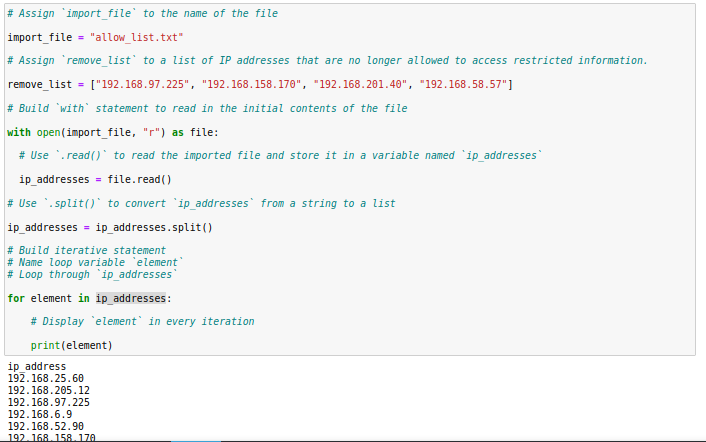
Remove IP addresses that are on the remove list
In order to remove the IP addresses from allow_list.txt that are on the remove list, we will use the .remove() in order to remove the value from our list:
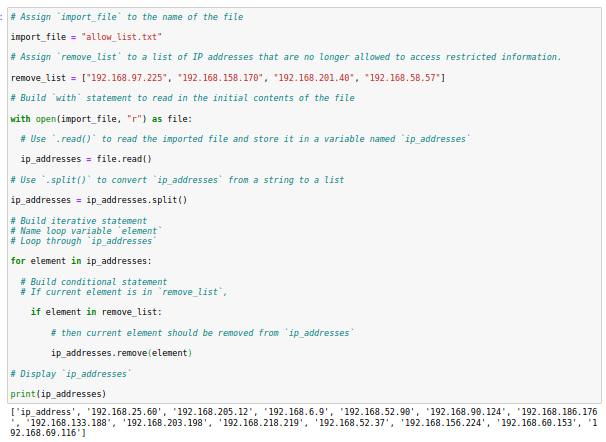
Update the file with the revised list of IP addresses
Now we update the file by opening it with write permissions and passing our new ip_address list value to the file.write function:
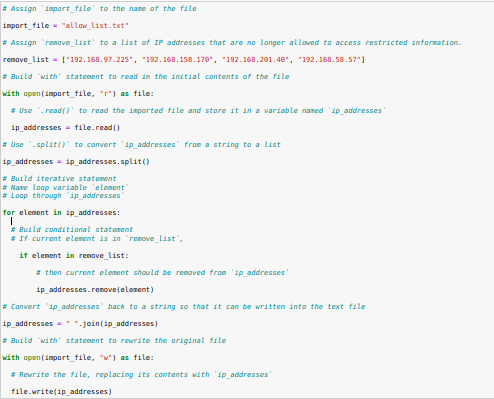
Then we verify that the file was overwritten:
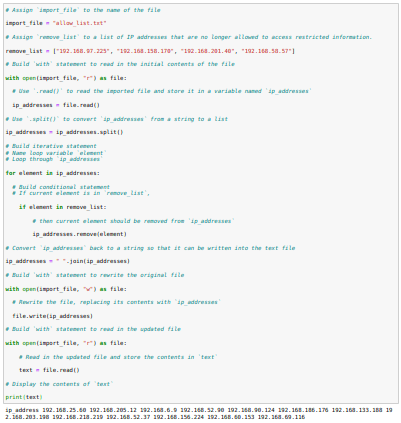
Summary
In this project, we created a Python script to:
- Open a file & get the file contents
- Compare that file’s contents with a list
- Edit the file contents based on the list comparison
- Verify changes